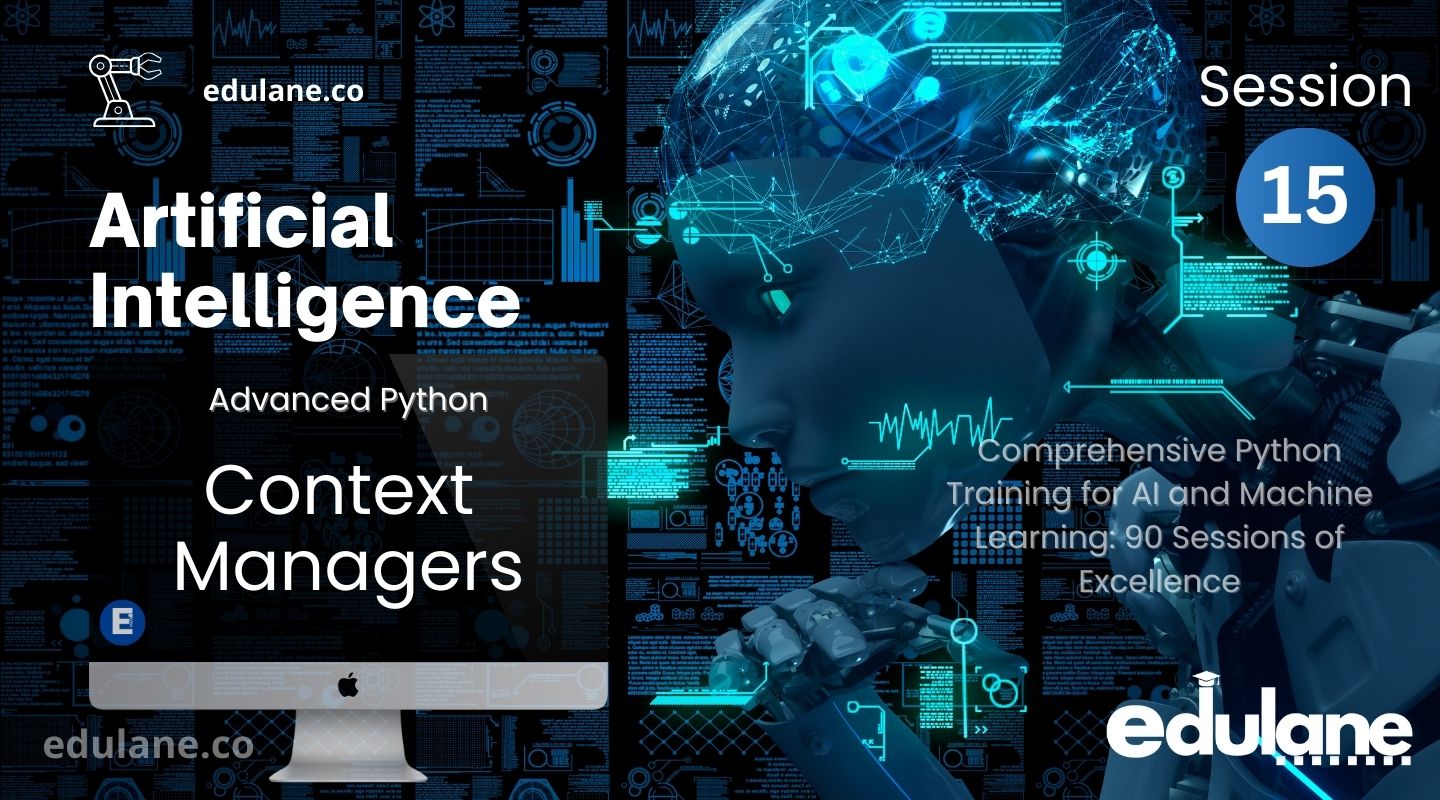
A context manager in Python is a way to allocate and release resources precisely when you need to. The most common use case for a context manager is file operations, but it can be used for many other tasks, like managing database connections, threading locks, etc.
Context managers are implemented using two methods: __enter__()
and __exit__()
. The with
statement in Python is used to wrap the execution of a block of code. The context manager sets up a context for the block of code and takes care of the cleanup after the block of code has been executed, even if an exception occurs.
Implementation of a Context Manager
Using a Class
class FileManager:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, exc_type, exc_val, exc_tb):
self.file.close()
if exc_type:
print(f"An exception occurred: {exc_val}")
return True # Suppress the exception if needed
# Usage
with FileManager('test.txt', 'w') as f:
f.write('Hello, World!')
In this example, FileManager
class handles the file operations. The __enter__()
method opens the file and returns it, while the __exit__()
method closes the file and optionally handles any exceptions.
Using a Decorator (contextlib
module)
from contextlib import contextmanager
@contextmanager
def file_manager(filename, mode):
f = open(filename, mode)
try:
yield f
finally:
f.close()
# Usage
with file_manager('test.txt', 'w') as f:
f.write('Hello, World!')
Here, the file_manager
function is decorated with @contextmanager
, which allows it to be used as a context manager. The code before the yield
statement is executed when entering the context, and the code after the yield
is executed when exiting the context.
Explanation
- Entering the Context (
__enter__
): When thewith
statement is executed, the__enter__()
method is called. It sets up the resource and returns it. The returned value is assigned to the variable after theas
keyword. - Executing the Block: The block of code under the
with
statement is executed. - Exiting the Context (
__exit__
): After the block of code is executed, the__exit__()
method is called. This method takes care of any cleanup actions like closing a file or releasing a lock.
Example: Custom Context Manager
class DatabaseConnection:
def __init__(self, db_name):
self.db_name = db_name
def __enter__(self):
print(f"Connecting to {self.db_name} database")
self.connection = self._connect_to_db()
return self.connection
def __exit__(self, exc_type, exc_val, exc_tb):
print(f"Closing connection to {self.db_name} database")
self.connection.close()
if exc_type:
print(f"An exception occurred: {exc_val}")
return True
def _connect_to_db(self):
# Simulating database connection
class Connection:
def close(self):
print("Connection closed")
return Connection()
# Usage
with DatabaseConnection('my_database') as conn:
print("Performing database operations")
In this example, DatabaseConnection
class manages a mock database connection. The __enter__()
method simulates opening the connection, and the __exit__()
method closes it, ensuring proper resource management.
Using context managers ensures that resources are properly managed, reducing the risk of resource leaks and making the code more readable and maintainable.