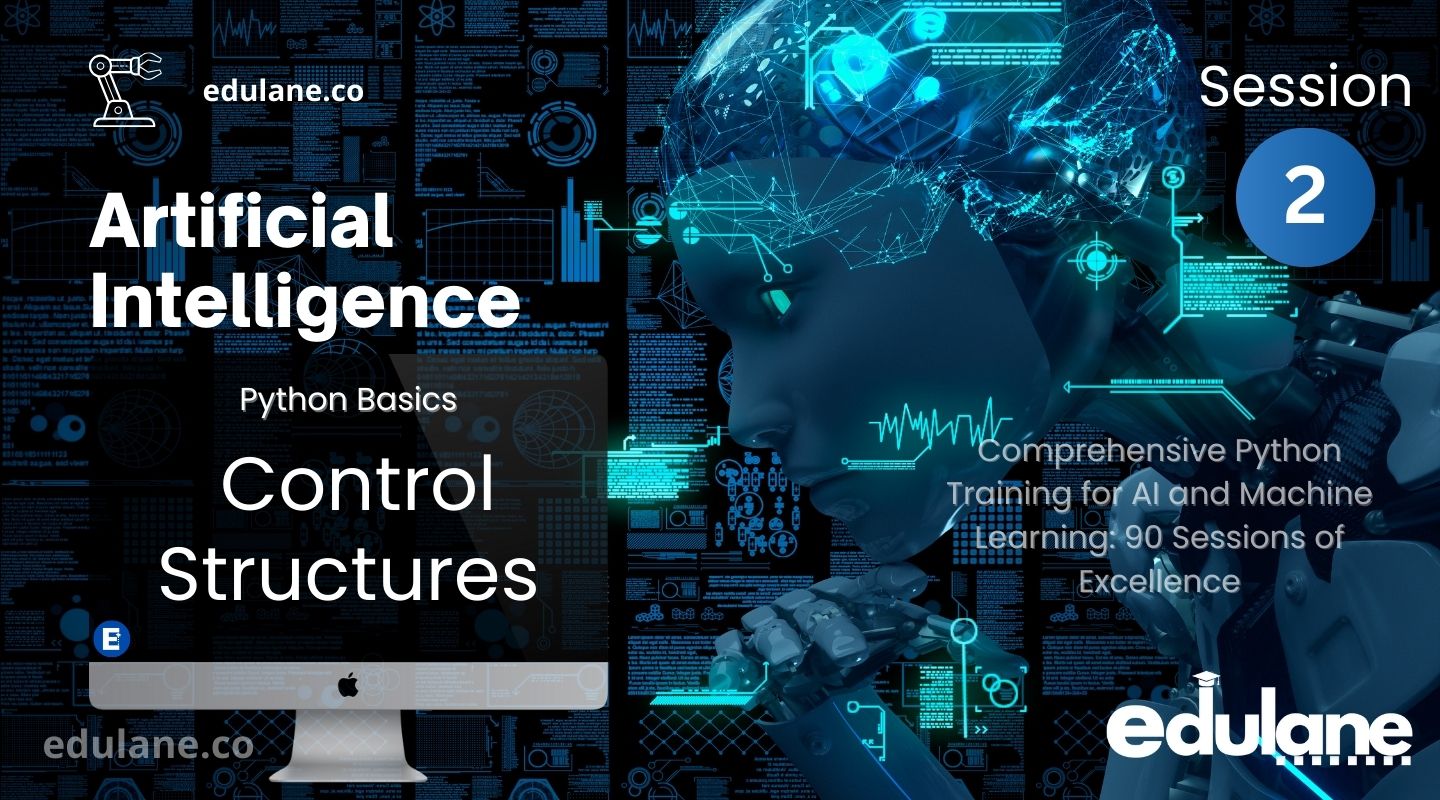
Section | Topic | Subtopics |
---|
1 | If Statements | Syntax, Example, Explanation |
2 | If-Else Statements | Syntax, Example, Explanation |
3 | Elif Statements | Syntax, Example, Explanation |
4 | Nested If Statements | Syntax, Example, Explanation |
5 | For Loops | Syntax, Example, Explanation |
6 | While Loops | Syntax, Example, Explanation |
7 | Break and Continue | Break Example, Continue Example, Explanation |
8 | List Comprehensions | Syntax, Example, Explanation |
9 | Dictionary Comprehensions | Syntax, Example, Explanation |
10 | Try-Except | Syntax, Example, Explanation |
11 | Best Practices | Readability, Comments, DRY Principle, Meaningful Names, PEP 8 |
12 | Complex Example: Nested Loops with Conditional Statements | Example, Explanation |
1. If Statements
The simplest form of control flow. It executes a block of code only if a condition is true.
Example:
age = 18
if age >= 18:
print("You are an adult.")
Output:
You are an adult.
Best Practices:
- Keep conditions simple and readable.
- Use comments to explain complex conditions.
2. if-else Statements
Allows you to execute one block of code if the condition is true and another if it’s false.
Example:
age = 16
if age >= 18
print("You are an adult.")
else:
print("You are a minor.")
Output:
You are a minor.
3. if-elif-else
Statements:
Handles multiple conditions.
Example:
age = 16
if age < 13:
print("You are a child.")
elif age < 18:
print("You are a teenager.")
else:
print("You are an adult.")
Output:
You are a teenager.
Best Practices:
- Ensure all possible conditions are covered to avoid unexpected behavior.
- Use
elif
to make the code more readable instead of nestedif-else
.
4. Nested If Statements:
Nested if statements allow you to check multiple conditions by placing one if
statement inside another if
statement. This can be useful for handling more complex decision-making scenarios.
Example:
number = 15
if number > 10:
if number % 2 == 0:
print("The number is greater than 10 and even.")
else:
print("The number is greater than 10 and odd.")
else:
print("The number is 10 or less.")
Output:
The number is greater than 10 and odd.
5. For Loops
Explanation: A for
loop iterates over a sequence (like a list, tuple, or string) and executes a block of code for each element in the sequence.
Example:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Output:
apple
banana
cherry
Example:
for i in range(3):
print("Hello, World!")
Output:
Hello, World!
Hello, World!
Hello, World!
Best Practices:
- Use meaningful variable names for loop variables.
- Avoid modifying the sequence you’re iterating over.
6. while
Loops
A while
loop repeats as long as a condition is true.
Example:
codecount = 1
while count <= 3:
print(count)
count += 1
Output:
1
2
3
Example 2:
password = ""
while password != "1234":
password = input("Enter the password: ")
print("Access granted.")
Output (after entering “1234”):
Enter the password: 0000
Enter the password: 1234
Access granted.
Best Practices:
- Ensure the condition will eventually become false to avoid infinite loops.
- Keep the loop logic simple and clear.
7. Loop Control Statements:
Explanation:
Control the flow of loops using break
and continue
.
break
: Exits the loop immediately.continue
: Skips the rest of the code inside the loop for the current iteration and moves to the next iteration.
Example with break
:
for i in range(10):
if i == 5:
break
print(i)
Output:
0
1
2
3
4
Example with continue
:
for i in range(10):
if i % 2 == 0:
continue
print(i)
Output:
0
1
2
3
4
Best Practices:
- Use
break
andcontinue
sparingly for clearer and more maintainable code. - Avoid deeply nested loops and conditions.
8. List Comprehensions
Explanation:
List comprehensions provide a concise way to create lists.
Example:
squares = [x**2 for x in range(1, 6)]
print(squares)
Output:
[1, 4, 9, 16, 25]
Example 2:
evens = [x for x in range(10) if x % 2 == 0]
print(evens)
Output:
[0, 2, 4, 6, 8]
Best Practices:
- Use list comprehensions for simple and readable code.
- Avoid overly complex comprehensions that can be hard to read.
9. Dictionary Comprehensions
Explanation:
Dictionary comprehensions create dictionaries in a concise way.
Example:
squares = {x: x**2 for x in range(1, 6)}
print(squares)
Output:
{1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
Example 2:
word_lengths = {word: len(word) for word in ["Python", "is", "awesome"]}
print(word_lengths)
Output:
{'Python': 6, 'is': 2, 'awesome': 7}
Best Practices:
- Use dictionary comprehensions for concise and readable code.
- Avoid complex comprehensions that reduce readability.
10. Try-Except:
The try-except
block in Python is used to handle exceptions (errors) that occur during the execution of a program. It allows the program to continue running even if an error occurs, providing a way to gracefully handle potential problems.
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero.")
Output:
Cannot divide by zero.