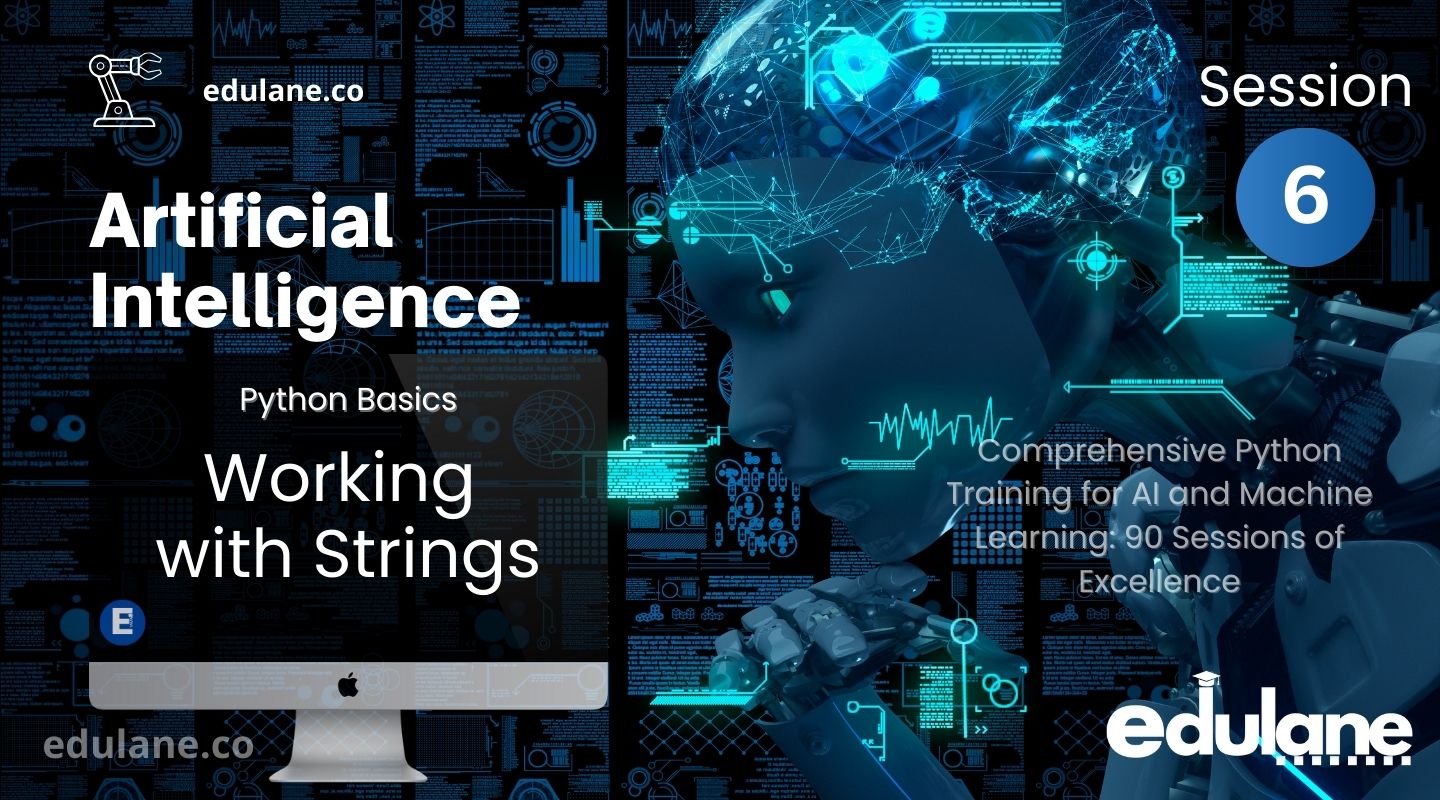
Table Of Content:
Basics of Python Strings:
In Python, strings are sequences of characters enclosed in single quotes ('
) or double quotes ("
). Here’s how you create strings:
# Single quotes
single_quoted = 'Hello, World!'
# Double quotes
double_quoted = "Hello, World!"
Accessing Characters:
Strings are indexed starting from 0. You can access characters using square brackets []
:
my_string = "Python"
print(my_string[0])
print(my_string[1])
Output:
'P'
'y'
String Length:
You can find the length of a string using the len()
function:
my_string = "Python"
print(len(my_string))
Output:
6
Concatenating Strings:
Strings can be concatenated using the +
operator:
string1 = "Hello"
string2 = "World"
concatenated_string = string1 + " " + string2
print(concatenated_string)
Output:
'Hello World'
String Methods:
Python strings have many built-in methods for manipulation:
my_string = " Hello, World! "
print(my_string.strip()) # Remove leading and trailing whitespace
print(my_string.lower()) # Convert to lowercase
print(my_string.upper()) # Convert to uppercase
print(my_string.replace("Hello", "Hi")) # Replace substring
print(my_string.split(",")) # Split string into a list
Output:
'Hello, World!'
' hello, world! '
' HELLO, WORLD! '
' Hi, World! '
[' Hello', ' World! ']
Advanced Concepts:
String Formatting:
Python offers several ways to format strings. Here’s an example using f-strings (formatted string literals):
name = "Alice"
age = 30
formatted_string = f"My name is {name} and I am {age} years old."
print(formatted_string)
Output:
'My name is Alice and I am 30 years old.'
Unicode Strings:
Python natively supports Unicode, allowing you to handle international characters:
unicode_string = "こんにちは"
print(unicode_string)
Raw Strings:
Raw strings treat backslashes (\
) as literal characters, useful for regular expressions and file paths:
raw_string = r'C:\path\to\file.txt'
print(raw_string)
Output:
C:\path\to\file.txt'
String Slicing:
You can slice strings to extract substrings.
my_string = "Python Programming"
print(my_string[0:6]) # Slice from index 0 to 5
print(my_string[-11:-1])
Output:
'Python'
'Programming'
Example:
# Example: Counting vowels in a string
def count_vowels(string):
vowels = "aeiouAEIOU"
count = 0
for char in string:
if char in vowels:
count += 1
return count
input_string = "Hello, how are you?"
print(f"The number of vowels in '{input_string}' is: {count_vowels(input_string)}")
Output:
The number of vowels in 'Hello, how are you?' is: 6
In this example:
- We define a function
count_vowels()
that counts vowels in a given string. - We use an input string
"Hello, how are you?"
. - Output shows the count of vowels in the input string.
This covers a range of string operations from basic manipulation to more advanced concepts like Unicode handling and string formatting.
Interview Questions:
Question 1: How do you create a string in Python?
Answer: Strings in Python are created by enclosing characters in single ('
) or double ("
) quotes.
Question 2: How can you access characters in a string?
Answer: Characters in a string can be accessed using indexing. For example, my_string[0] accesses the first character.
Question 3: How do you find the length of a string in Python?
Answer: Strings can be concatenated using the + operator.